Getting Started with SvelteKit
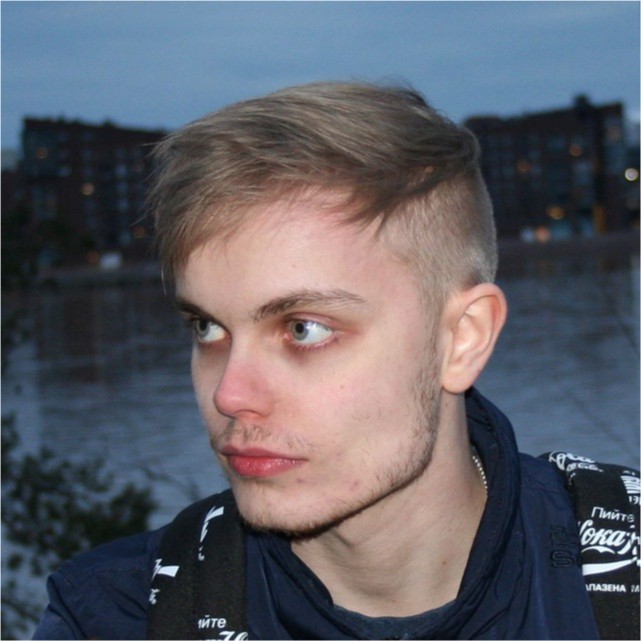
Aleksi Koivu
Founder -- KoivuDev
November 28, 2023
SvelteKit is a powerful web framework built on top of Svelte, designed to streamline the development of web applications. With its intuitive syntax, automatic code splitting, and serverless deployment capabilities, SvelteKit provides an efficient and enjoyable development experience. This article will guide you through the process of getting started with SvelteKit, covering installation, project structure, and basic concepts.
Prerequisites
Before diving into SvelteKit, ensure that you have Node.js and npm (Node Package Manager) installed on your machine. You can download them from nodejs.org.
Installation
To create a new SvelteKit project, open your terminal and run the following commands:
npx create-svelte@latest my-sveltekit-app
cd my-sveltekit-app
npm install
This will scaffold a new SvelteKit project and install its dependencies.
Project Structure
SvelteKit follows a convention-based project structure that organizes your code in a clear and modular way. Here’s an overview of the important directories:
src
: This directory contains your application’s source code.routes
: Define your application routes using Svelte files. Each file in this directory corresponds to a specific route.lib
: Place utility functions or shared logic here.components
: Store reusable Svelte components.
static
: Static assets such as images, stylesheets, and fonts go here.public
: Files in this directory will be accessible from the root of your deployed application.
Creating Your First Route
Let’s create a simple “Hello, SvelteKit!” route to get started. Open the src/routes/index.svelte
file and replace its content with the following:
<script>
export let name = 'SvelteKit';
</script>
<main>
<h1>Hello, {name}!</h1>
</main>
<style>
main {
text-align: center;
padding: 1em;
max-width: 240px;
margin: 0 auto;
}
h1 {
color: #ff3e00;
text-transform: uppercase;
font-size: 2em;
font-weight: 900;
margin: 0;
}
</style>
This Svelte component defines a simple greeting message with a dynamically rendered name. The <script>
, <main>
, and <style>
tags encapsulate the JavaScript logic, HTML structure, and CSS styles, respectively.
Running Your SvelteKit App
Now that you’ve created your first route, let’s run the application. In the terminal, navigate to your project’s root directory and run:
npm run dev
This command starts a development server, and you can access your SvelteKit app at http://localhost:5000
. Open this URL in your browser to see the “Hello, SvelteKit!” message.
Conclusion
Congratulations! You’ve successfully set up a SvelteKit project, created a basic route, and run your application locally. This is just the beginning; SvelteKit offers many more features to explore, such as layouts, stores, and serverless deployment options. Refer to the official documentation for more in-depth information and advanced usage. Happy coding with SvelteKit!